TechFreak
Tuesday, March 31, 2015
Wednesday, September 24, 2014
Create a simple blog using Laravel 4
In this article we will create a simple blog using Laravel 4. Our blog application will have the following features:
- Display posts with read more links on home page.
- Users will able to search posts on blog.
- Display single post with comments.
- Allow users to post comments.
- Administrator will be able to perform CRUD operations on posts and comments.
- Administrator will be able to moderate comments.
Laravel Quick Installation and Setup
I will assume that you have a working installation of Laravel 4. Otherwise, you can follow these steps to install and setup Laravel 4:
- Install Laravel 4 by following instructions provided here.
- Create a database using the MySQL terminal client:
┌─[usm4n@usm4n-desktop]―[~] └─•mysql -u root -p Enter password: mysql> create database laravel; Query OK, 1 row affected (0.00 sec)
- Configure database in /app/config/database.php:
- 'mysql' => array(
- 'driver' => 'mysql',
- 'host' => 'localhost',
- 'database' => 'laravel',
- 'username' => 'root',
- 'password' => 'very_secret_password',
- 'charset' => 'utf8',
- 'collation' => 'utf8_unicode_ci',
- 'prefix' => '',
- ),
Creating Database Migrations
In this section, we will create database tables for our blog application using Laravel Database Migrations. Our application will be utilizing
posts
and comments
tables to store articles and user comments respectively. (Read more on migrations here)QUICK TIP:
We useartisan migrate:make create_tablename_table
andartisan migrate
commands to create and run migrations respectively.
Migration
class
for posts
table:
- <?php
- use Illuminate\Database\Migrations\Migration;
- use Illuminate\Database\Schema\Blueprint;
- class CreatePostsTable extends Migration {
- /**
- * Run the migrations.
- *
- * @return void
- */
- public function up()
- {
- Schema::create('posts', function(Blueprint $table) {
- $table->increments('id');
- $table->string('title');
- $table->string('read_more');
- $table->text('content');
- $table->unsignedInteger('comment_count');
- $table->timestamps();
- $table->engine = 'MyISAM';
- });
- DB::statement('ALTER TABLE posts ADD FULLTEXT search(title, content)');
- }
- /**
- * Reverse the migrations.
- *
- * @return void
- */
- public function down()
- {
- Schema::table('posts', function(Blueprint $table) {
- $table->dropIndex('search');
- $table->drop();
- });
- }
- }
Note that, I have used
$table->engine='MyISAM'
and added a composite fulltext index usingtitle
and content
columns. This will allow us to utilize MySQL fulltext search on posts table.
Code for
comments
table migration:
- <?php
- use Illuminate\Database\Migrations\Migration;
- use Illuminate\Database\Schema\Blueprint;
- class CreateCommentsTable extends Migration {
- /**
- * Run the migrations.
- *
- * @return void
- */
- public function up()
- {
- Schema::create('comments', function(Blueprint $table) {
- $table->increments('id');
- $table->unsignedInteger('post_id');
- $table->string('commenter');
- $table->string('email');
- $table->text('comment');
- $table->boolean('approved');
- $table->timestamps();
- });
- }
- /**
- * Reverse the migrations.
- *
- * @return void
- */
- public function down()
- {
- Schema::drop('comments');
- }
- }
The
post_id
field will help us in defining one to many relationship using Eloquent ORM seamlessly. We will use approved
field of comments table for comments moderation purpose. We will cover the users
table in Authentication Section.Creating Models Using Eloquent ORM
The Eloquent ORM included with Laravel provides a beautiful, simple ActiveRecord implementation for working with your database. Each database table has a corresponding “Model” which is used to interact with that table.Laravel Eloquent ORM Documentation
We use singular variants of table names as our Eloqent Model names, this convention helps the Eloquent to figure out the table to be used with a particular Model. For instance: if the name of the Model is
Post
Eloquent will assume a table with name posts
to be used.
Here is the code for our
Post
and Comment
Models:
- <?php
- // file: app/models/Post.php
- class Post extends Eloquent {
- public function comments()
- {
- return $this->hasMany('Comment');
- }
- }
- // file: app/models/Comment.php
- class Comment extends Eloquent {
- public function post()
- {
- return $this->belongsTo('Post');
- }
- }
Seeding Database Tables
We will use a single
PostCommentSeeder
class to populate both posts
and comments
tables.QUICK TIP:
We useartisan db:seed
command to seed the database.
Code for
PostCommentSeeder
:
- <?php
- class PostCommentSeeder extends Seeder {
- public function run()
- {
- $content = 'Lorem ipsum dolor sit amet, consectetur adipiscing elit.
- Praesent vel ligula scelerisque, vehicula dui eu, fermentum velit.
- Phasellus ac ornare eros, quis malesuada augue. Nunc ac nibh at mauris dapibus fermentum.
- In in aliquet nisi, ut scelerisque arcu. Integer tempor, nunc ac lacinia cursus,
- mauris justo volutpat elit,
- eget accumsan nulla nisi ut nisi. Etiam non convallis ligula. Nulla urna augue,
- dignissim ac semper in, ornare ac mauris. Duis nec felis mauris.';
- for( $i = 1 ; $i <= 20 ; $i++ )
- {
- $post = new Post;
- $post->title = "Post no $i";
- $post->read_more = substr($content, 0, 120);
- $post->content = $content;
- $post->save();
- $maxComments = mt_rand(3,15);
- for( $j = 1 ; $j <= $maxComments; $j++)
- {
- $comment = new Comment;
- $comment->commenter = 'xyz';
- $comment->comment = substr($content, 0, 120);
- $comment->email = 'xyz@xmail.com';
- $comment->approved = 1;
- $post->comments()->save($comment);
- $post->increment('comment_count');
- }
- }
- }
- }
The outer for loop creates a new
Post
Model on each iteration and saves it after setting the properties: title
, read_more
, content
. The inner loop creates random number of comments for each Post
and increments the comment_count
in posts
table.The ‘artisan tinker’ Command
The Artisan CLI tool provides us with an easy way to interact with Laravel application from command line. We use
artisan tinker
command to use the interactive shell. Let’s practice some Eloquent queries on our test data.┌─[usm4n@usm4n-desktop]―[~] └─•artisan tinker >
Find By Id Using find()
>$post = Post::find(2); >$post->setHidden(['content','read_more','updated_at']); >echo $post; {"id":"2","title":"Post no 2","comment_count":"7","created_at":"2014-01-06 09:43:44"}
Limit No Of Records Using take() And skip()
>$post = Post::skip(5)->take(2)->get(); >foreach($post as $value) echo "post id:$value->id "; post id:6 post id:7
Using select() And first()
>$post = Post::select('id','title')->first(); >echo $post; {"id":"1","title":"Post no 1"}
Using where() With select()
>$post = Post::select('id','title')->where('id','=',10)->first(); >echo $post; {"id":"10","title":"Post no 10"}
Getting Related Records Using Dynamic Property
>$post = Post::find(4); >echo $post->comments[0]->commenter; xyz
Getting Parent Records From Child Model
>$comment = Comment::find(1); >echo $comment->post->title; Post no 1
For a complete reference on Eloquent ORM queries visit official Eloquent Documentation Page.
That’s all for now. Thanks and Regards!
Tuesday, July 22, 2014
An Introduction about Laravel Framework
Introduction
Laravel is a very powerful framework, designed for php web application. It will give you ways to modularize your codes and make them look cleaner and easier to read and understand. It is open source software under MIT License.
Features :
Now, we will talk about some prominent features of this framework which make it different from other :
Bundles : These are small packages which you may download to add certain functionality to your web application. Hence, saving a lot of coding stuff and time. These may be containing some custom functionality.
Class Autoloading: It assure that correct components are loaded at correct time and saves a lot of your headache.
View Composer : Theses are block of codes which can run when view is loaded.
Unit testing: Laravel allows user to easily create unit test and run these test making use of Artisan utility.The best thing is it itself supports a number of test to ensure application stability.
The Eloquent ORM: The Eloquent ORM is the most advanced PHP ActiveRecord implementation available. With the capacity to easily apply constraints to both relationships and nested eager-loading you'll have complete control over your data with all of the conveniences of ActiveRecord.
Application Logic : can be implemented within your application either using controllers or directly into route declarations.
Reverse Routing : allows you to create links to named routes. When creating links just use the route's name and Laravel will automatically insert the correct URI. This allows you to change your routes at a later time and Laravel will update all of the relevant links site-wide.
Restful Controllers: are an optional way to separate your GET and POST request logic. In a login example your controller's get_login() action would serve up the form and your controller's post_login() action would accept the posted form, validate, and either redirect to the login form with an error message or redirect your user to their dashboard.
The IoC container (Inversion of Control): Inversion of Control gives you a method for generating new objects and optionally instantiating and referencing singletons. IoC means that you'll rarely ever need to bootstrap any external libraries. It also means that you can access these objects from anywhere in your code without needing to deal with an inflexible monolithic structure.
Installation and Setup of Laravel
Requirements:
- Apache or any other compatible web server
- Since, Laravel makes use of PHP 5.3 features so it’s also requirement.
- For detecting file Mime type Laravel make use of FileInfo Library. Windows users must enable this by making appropriate changes(include the bundled php_fileinfo.dll DLL file in php.ini) to include this extension.
- It make use of Mcrypt library for security purposes. This library is mostly preinstalled but if you have problems in finding this, then check the vendor site of your LAMP installation
Installation
- Download Laravel from http://laravel.com/download
- Extract the Laravel archive and upload the contents to your web server.
- Set the value of the key option in the config/application.php file to a random, 32 character string.
- Verify that the storage/views directory is writable.
- Navigate to your application in a web browser.
Personally, I used Wamp server which included everything needed. This made every requirements fulfillment too easy. You can directly include extensions by making use of GUI.
If everything is fine you must see a welcome page like below :
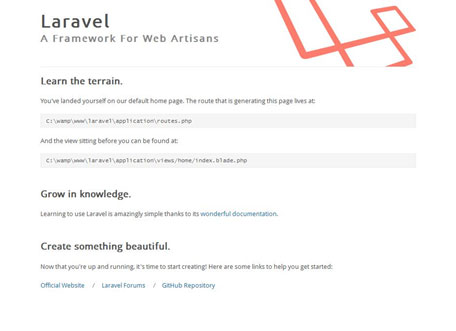
Figure 1: Welcome Page
Server Configuration
Laravel tends to protect your codes, bundles by keeping only those files that are necessarily public in the web server's DocumentRoot. This prevents server misconfiguration from getting your codes leaked or accessible to third party.
Basic configuration
All of the configurations provided are located in your applications config/ directory.Make sure you change the 32 character key inside the application.php inside the config folder. Make the key as any random 32 character string. You may automatically generate key making use of Artisan command utility, which we will discuss later.
Environment
Its most likely that the configuration you set for local environment is not same as production. Laravel default environment handling mechanism is URL based, which will make setting up environments very easy. If you open the paths.php from your Laravel installation root directory, you will see the below:
Listing 1: Paths.php
$environments = array( 'local' => array('http://localhost*', '*.dev'), );
Here, all the url which are starting with localhost and ending with.dev will be considered under local environment. Now if you create a directory local inside application/config then it will override the options in the base application/config directory. You may create as many environment as you like.
Key terms
Here are some key term which you must be knowing :
Models : Models are the heart of your application. It mainly consist of Business Logic. The models mainly comprises of Database interactions, File I/O operations ,Interacting with web services etc.
Libraries : Libraries are classes that perform tasks that aren't specific to your application. For example a library which can convert your table data to graphs and show that. Creating library is too easy. For eg we create a ShowMess.php with following content and place this inside the library folder:
Listing 2: Library
<?php class ShowMess { public static function write($text) { echo $text; } }
Now we can call ShowMess::write(“Displaying of text making use of library. This is too simple and easy”) from anywhere in our application which will print “Displaying of text making use of library. This is too simple and easy”.
With Laravel Auto loading feature it is very easy to use both Models and Library.
Troubleshooting
In case of installation problems please check the below points:
- Make sure the public directory is document root of your web server.
- If you are using mod_rewrite, set the index option in application/config/application.php to an empty string.
- Verify that your storage folder and the folders within are writable by your web server.
This is all about an introduction to Laravel. In coming tutorials we will learn a lot about making use of this exciting framework in our web application. Hope you liked the article, see you next time with some more informational article.
Monday, August 5, 2013
Nvidia Shield benchmarked and torn down: Is this the first and last Tegra 4 device?
Yesterday, Nvidia’s Shield handheld game console was finally released to the public — and today, it has been torn down and benchmarked, revealing impressive performance figures for the Tegra 4′s first outing. The first batch of Shield reviews were generally mediocre, citing a high price and limited games library, but they did all agree on one positive point: The crippling lack of functionality aside, the hardware itself is seriously impressive.
The Android-powered Shield comes with a quad-core Tegra 4 SoC (four Cortex-A15 cores, plus a fifth low-power Cortex-A15 “companion core” that performs background tasks), a 72-core GPU, 5-inch 1280×720 display, 16GB of storage, and 2GB of RAM. There’s also a couple of speakers that reviewers are generally very complimentary about, and the usual WiFi and Bluetooth connectivity. Benchmark-wise, Tegra 4 is the currently the fastest Android device on the market, beating out Qualcomm’s Snapdragon 800 — though the iPad 4′s GPU still beats the Tegra 4 GPU in some tests.
Due to its much larger form factor, though, it doesn’t really make sense to compare the Shield’s performance to smartphones and tablets. Where the Shield has a 28.8 watt-hours battery — and a fan! — to keep the power-hungry Cortex-A15 cores fed, a smartphone battery might only have a capacity of 6 watt-hours. It’s very rare for ARM-powered smartphones and tablets to have a fan, too. As a result, the Shield can run its CPU and GPU at much higher clock speeds — and thus post some impressive benchmark scores.
The teardown, of course, is courtesy of iFixit. Inside the Shield (which is around the same size as an Xbox 360 gamepad but twice as heavy), there is a main logic board, three huge batteries, another board that manages all of the gamepad inputs, and the screen. There aren’t really any surprises here; it’s basically just a high-powered portable gaming machine. The Tegra 4 SoC (28nm) is around the same size as the Tegra 3 (40nm), with the extra space granted by the die shrink being gobbled up by the larger CPUs and GPU. It is amusing to see a fan, though; those Cortex-A15 cores must really be drawing a significant amount of power. The motherboard also provides the micro SD, mini HDMI, micro USB, and 3.5mm headphone connectors.
Overall, iFixit gives the Shield a repairability score of 6 out of 10, losing points due to a tricky-to-replace battery and display.
While the hardware is impressive, then, it’s a shame that the device as a whole leaves much to be desired. The fact that there are very few good games for the Shield, coupled with its exorbitant price point ($300), makes it very hard to recommend the Shield as a handheld gaming machine. Game streaming from your PC to the Shield is an exciting prospect, but it only works on your local network; the PS4, which costs just $100 more, will be able to stream games across the internet with Remote Play. You could possibly make the argument that the Shield makes for a high-powered smartphone or tablet replacement, but the fact that it doesn’t contain a cellular modem, is cumbersome to hold in one hand, and doesn’t fit in your pocket precludes this.
The bigger question, of course, is whether we’ll actually see the Tegra 4 SoC in more than a handful of devices. Its performance can obviously competitive in the right setting — but if it needs a huge battery and a fan, then it’s easy to see why smartphone and tablet makers have almost universally opted for Qualcomm SoCs with its Krait CPU cores, rather than the SoCs based on the Cortex-A15 CPU.
Subscribe to:
Posts (Atom)